Missing Authorization header in Angular 7 HTTP response
Accessing an API back-end from Angular client may result in missing response headers. A common scenario is a missing Authorization header, containing the JSON Web Token (JWT) which is returned from the back-end service when the user logs in successfully. The solution to the problem is to expose the desired header in the back-end code (notice that the Authorization header is not exposed by default). In the case of a Spring Boot back-end, we need to add the following line of code:
response.addHeader("Access-Control-Expose-Headers", "Authorization");
@Override
protected void successfulAuthentication(HttpServletRequest request, HttpServletResponse response,
FilterChain chain, Authentication authResult) throws IOException, ServletException {
String token = JWT.create()
.withSubject(((User) authResult.getPrincipal()).getUsername())
.withExpiresAt(new Date(System.currentTimeMillis() + EXPIRATION_TIME))
.sign(HMAC512(SECRET.getBytes()));
response.addHeader("Access-Control-Expose-Headers", "Authorization");
response.addHeader(HEADER_STRING, TOKEN_PREFIX + token);
}
Letzte Beiträge
Share :
Share :
Weitere Beiträge
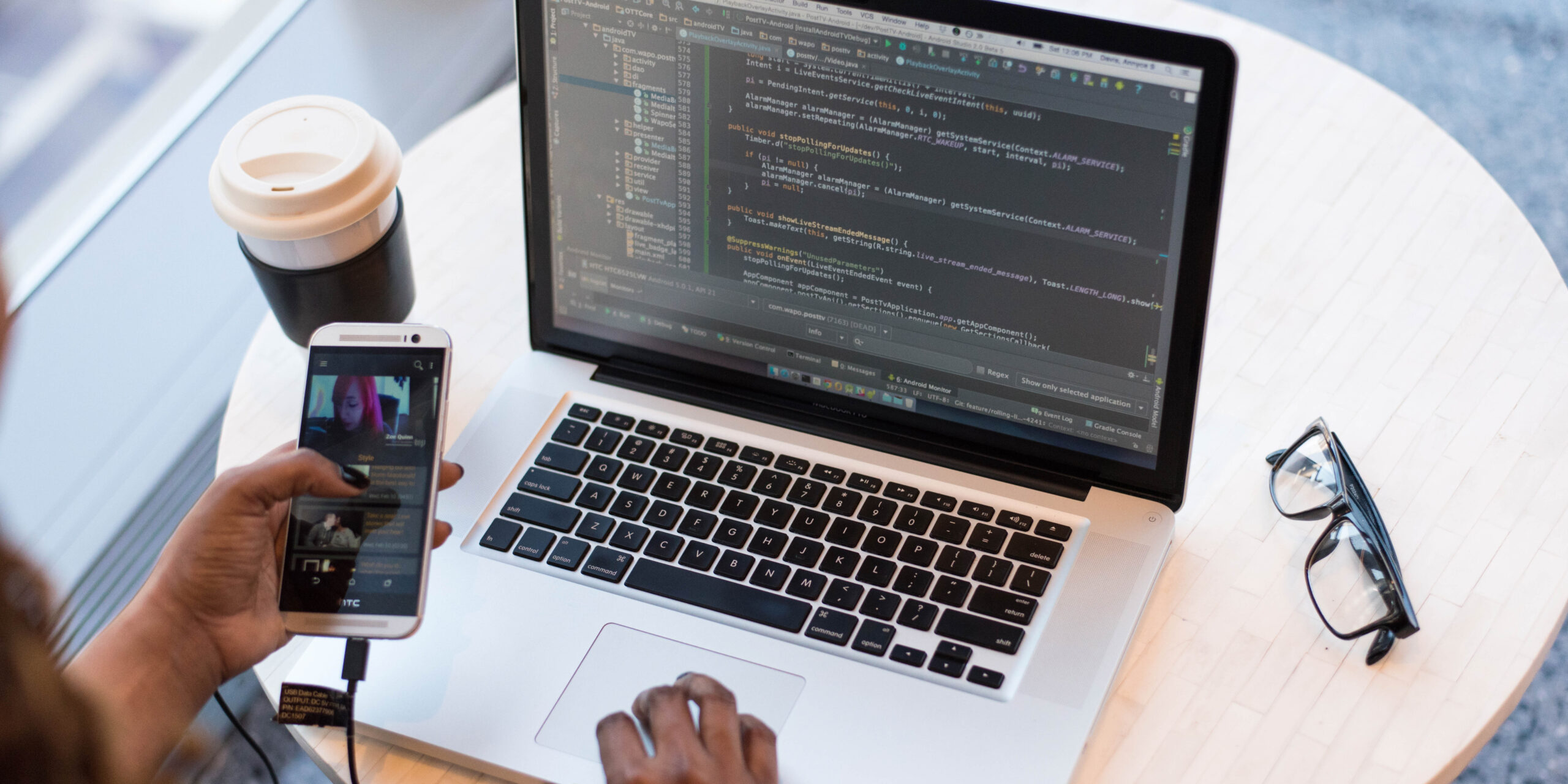
LiveData
LiveData is an observable data holder class. It is also lifecycle-aware, which means that it respects the lifecycle of the other app components, such as activities, fragments and services. It notifies only active observers, represented by the Observer class.
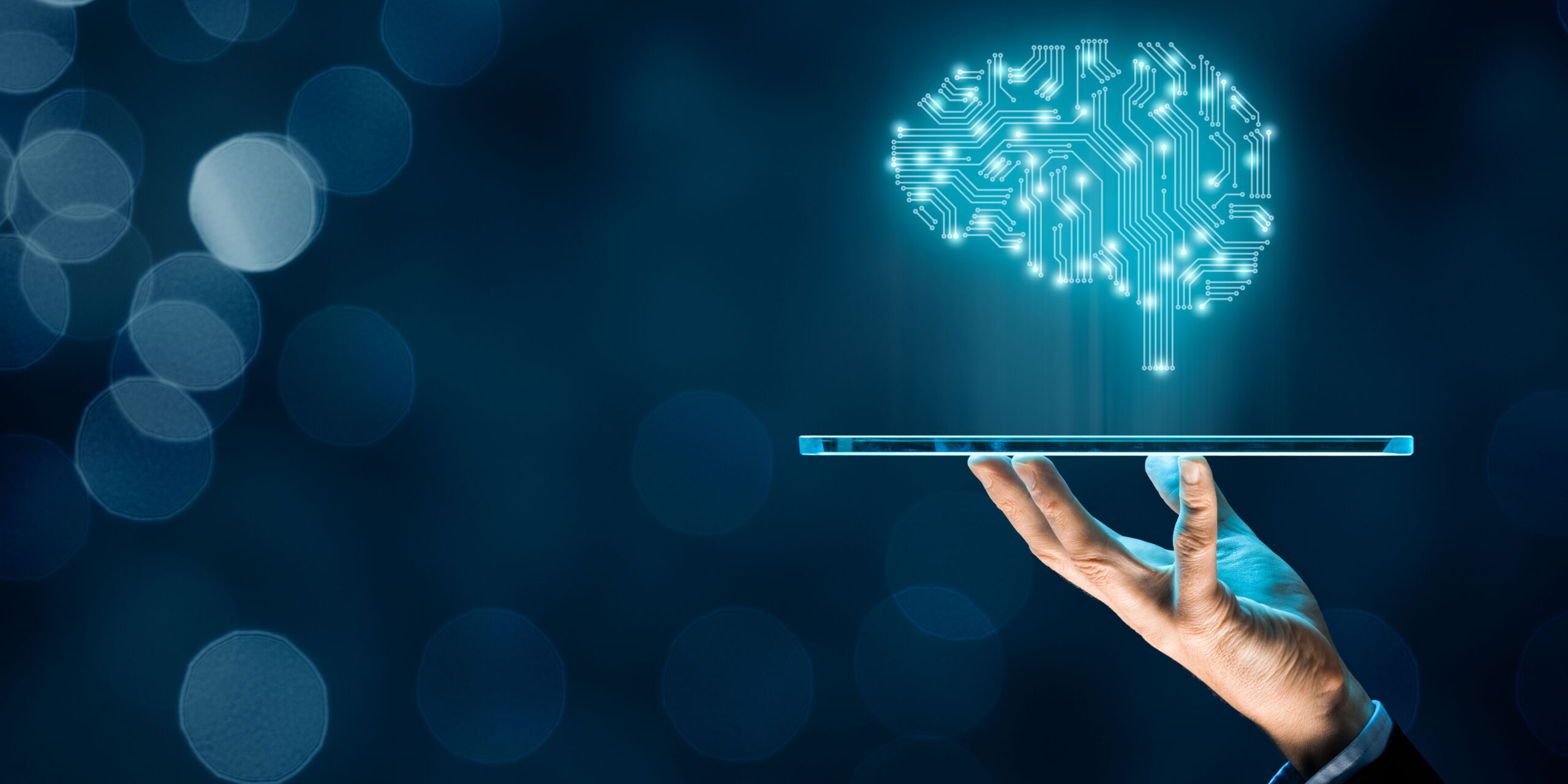
Machine learning concepts. Data preparation
Each machine learning process related to the use of neural networks consists of at least two parts. The first part is related to data loading and preparation for training.
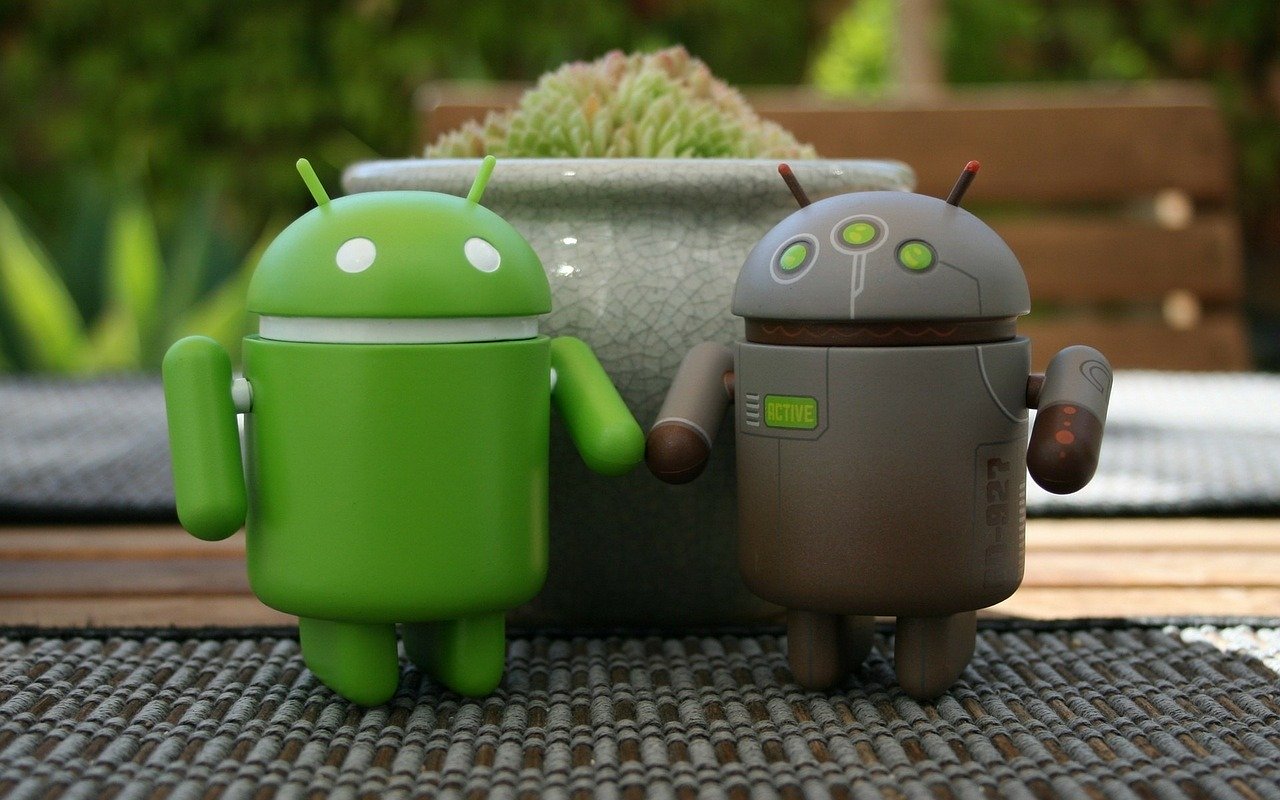
MVVM’s Model implementation in Android application. Room
As we said in the previous article Model is the data in our application. These are classes representing objects that we persist in our database or that we get from network calls to services.