Dependency Injection
What is Dependency Injection?
Each Object-oriented application consists of many classes that work together in order to solve a problem. However, when writing a complex application, application classes should be as independent as possible. DI helps in gluing these classes together and at the same time keeping them independent. Dependency injection is a technique whereby one object (or static method) supplies the dependencies of another object.
Pros
- increase the possibility to reuse classes
- test them independently of other classes while unit testing
Code with no DI vs code with DI
public class Computer {
private CPU cpu;
public Computer() {
this.cpu = new CPU();
}
}
Dependency between Computer and CPU.
public class Computer {
private CPU cpu;
public Computer(CPU cpu) {
this.cpu = cpu;
}
}
Dependency between Computer and CPU. Dependency is injected through class constructor (Computer should not worry about CPU implementation).
Types of DI
- Constructor-based dependency injection – used for mandatory dependencies
public Computer(CPU cpu) {
this.cpu = cpu;
}
- Setter-based dependency injection – used for optional dependencies
public Computer(CPU cpu) {
this.cpu = cpu;
}
- Property-based dependency injection – considered as bad practice!
@Autowired
private CPU cpu;
Letzte Beiträge
Share :
Share :
Weitere Beiträge
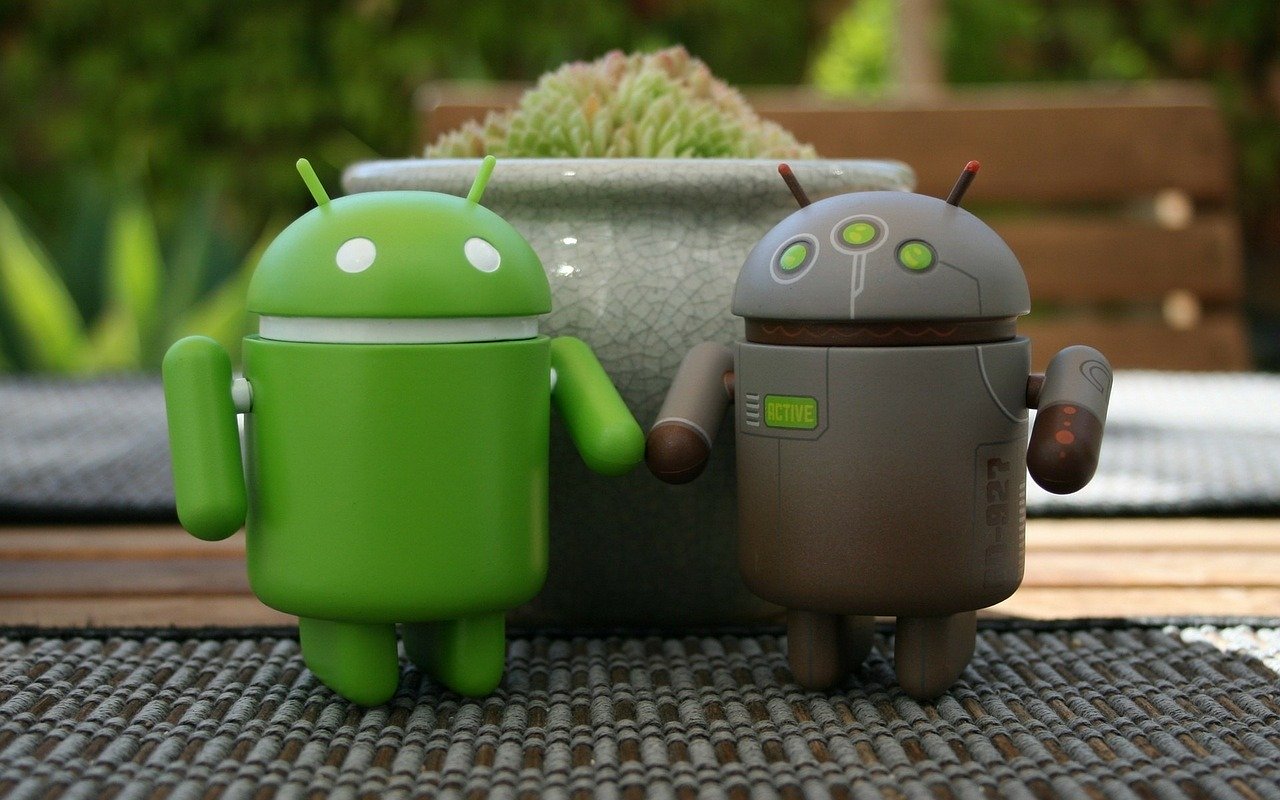
MVVM’s Model implementation in Android application. Room
As we said in the previous article Model is the data in our application. These are classes representing objects that we persist in our database or that we get from network calls to services.
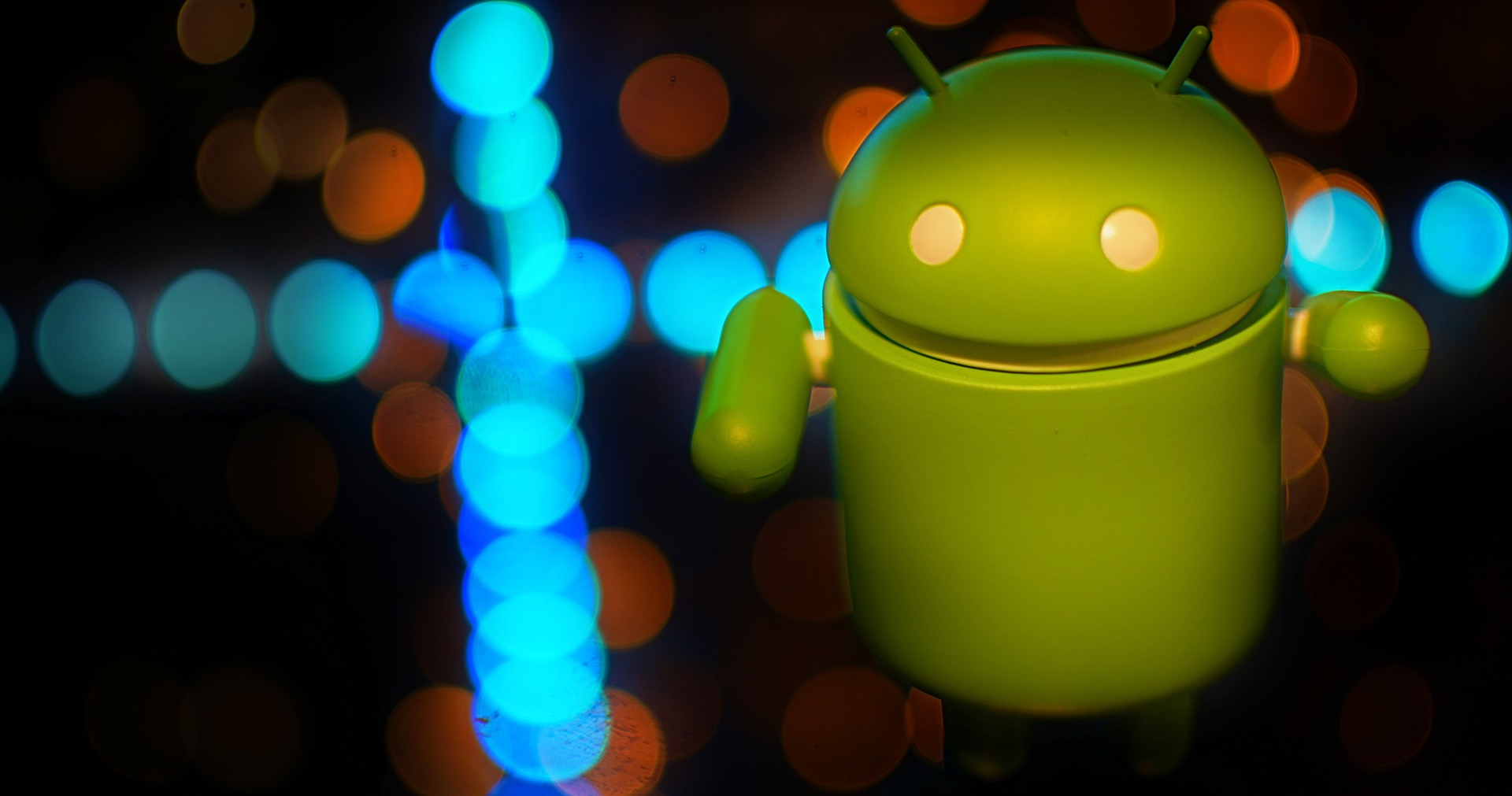
Repository in Android’s MVVM architecture
Repository is a class which purpose is to provide a clean API for accessing data. What that means is that the Repository can gather data from different data sources(different REST APIs, cache, local database storage) and it provides this data to the rest of the app.
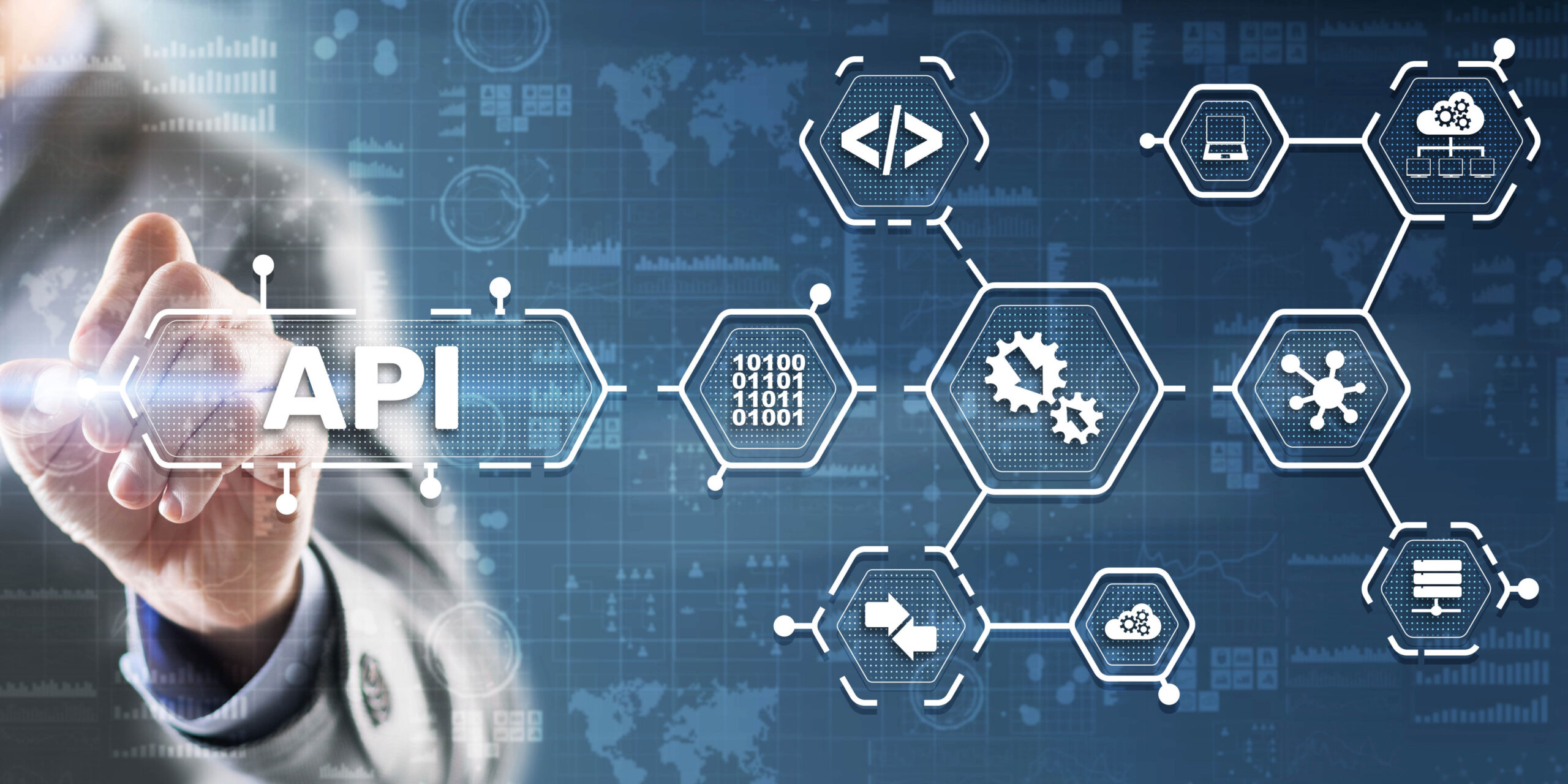
Missing Authorization header in Angular 7 HTTP response
Accessing an API back-end from Angular client may result in missing response headers. A common scenario is a missing Authorization header, containing the JSON Web Token (JWT) which is returned from the back-end service when the user logs in successfully.