Handling Events with Lambda Expressions
Lambda Expressions and Functional Interfaces are a new feature of Java 8 and the support provided for lambda expressions is only with functional interfaces. A functional interface is an interface that contains only one abstract method. This is exactly the case with handling events in Java and more accurately for these examples for handling events using the JavaFX library. Prior to lambda expressions the code execution for a button click would go this way:
button.setOnAction(new EventHandler() {
@Override
public void handle(ActionEvent actionEvent) {
String newColor = "rgba(100, 0, 100)";
stackPane.setStyle("-fx-background-color: " + newColor);
}
});
Here is created an anonymous class that implements the EventHandler interface and it is being passed to the method setOnAction. The code that will be executed whenever the button is fired is placed within the handle method which is the only method in the EventHandler interface which makes it a functional interface. With lambda expressions the code would look like this:
button.setOnAction((ActionEvent event) -> {
String newColor = "rgba(100, 100, 0)";
stackPane.setStyle("-fx-background-color: " + newColor);
});
In this case the compiler can infer the name of the method because it is the only method in the interface so the body and the parameter declaration is enough.
Syntax
Best Practices
Lambda expressions should be simplified as much as possible. For example the event type declaration from the previous example code can be removed because the EventHandler expects an ActionEvent and the type of the event reference can also be inferred making the code like this:
button.setOnAction((event) -> {
String newColor = "rgba(100, 100, 0)";
stackPane.setStyle("-fx-background-color: " + newColor);
});
Because parentheses are not mandatory when there is one parameter passed, another improvement would be to remove them as well:
button.setOnAction(event -> {
String newColor = "rgba(100, 100, 0)";
stackPane.setStyle("-fx-background-color: " + newColor);
});
If there is only one line of code with a return statement in the method body, the return statement can also be removed.
“Effectively Final” Variables
public void start(Stage primaryStage) {
String color = "rgba(0, 100, 100)";
Trying to assign a value to color will pop-up the message: “Variable ‘color’ is already defined in the scope.”
button.setOnAction((ActionEvent event) -> {
String color = "rgba(100, 100, 0)";
stackPane.setStyle("-fx-background-color: " + color);
});
Running it will produce the error:
Error:(33,20) java: variable color is already defined in method start(javafx.stage.Stage)
Letzte Beiträge
Share :
Share :
Weitere Beiträge
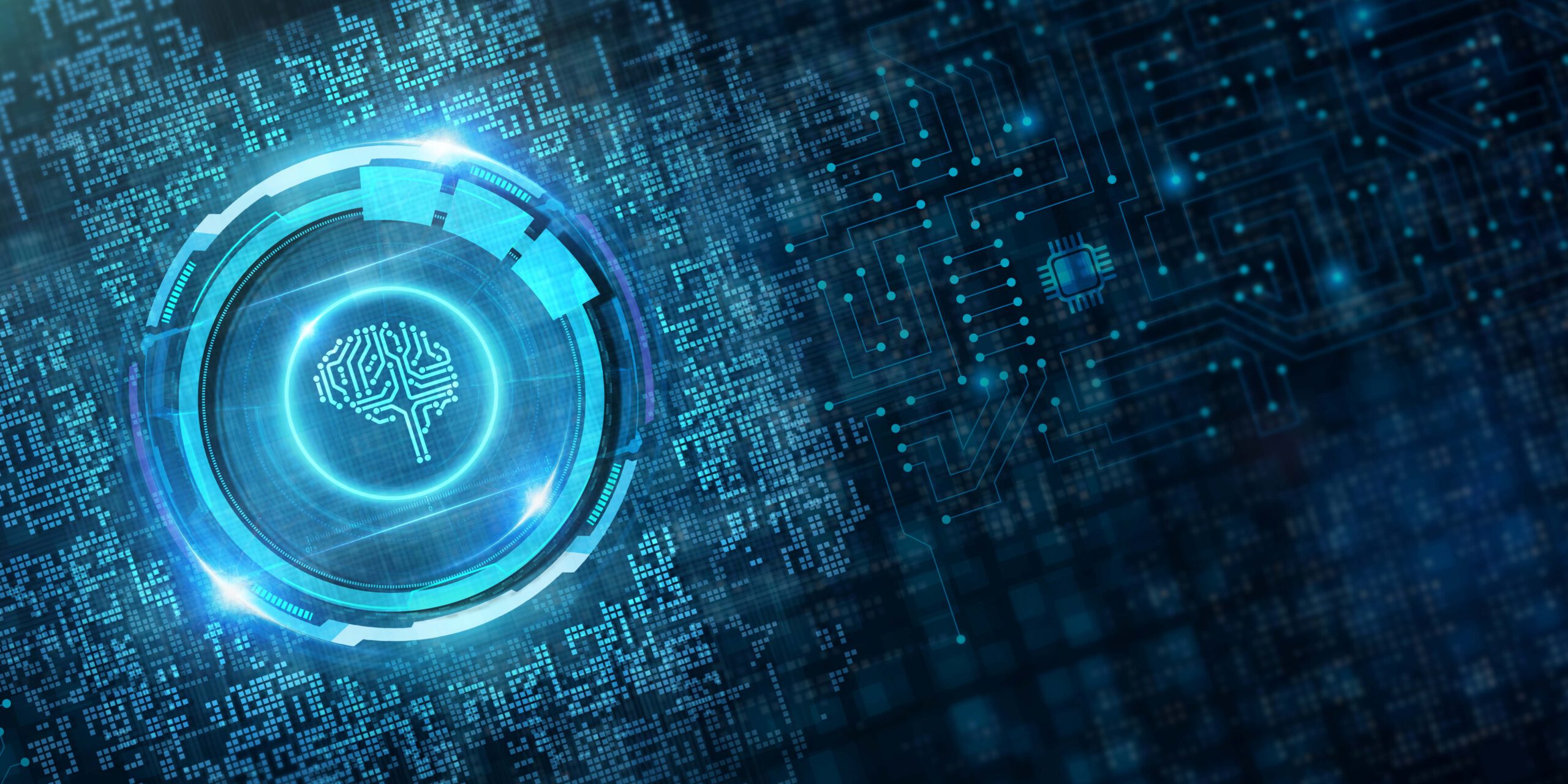
Dependency Injection with Spring 5
As we learned in the previous chapter dependency injection is a very powerful technique. DI is provided by the Spring 5 Framework implementation of the Inversion of Control (IoC) principle.
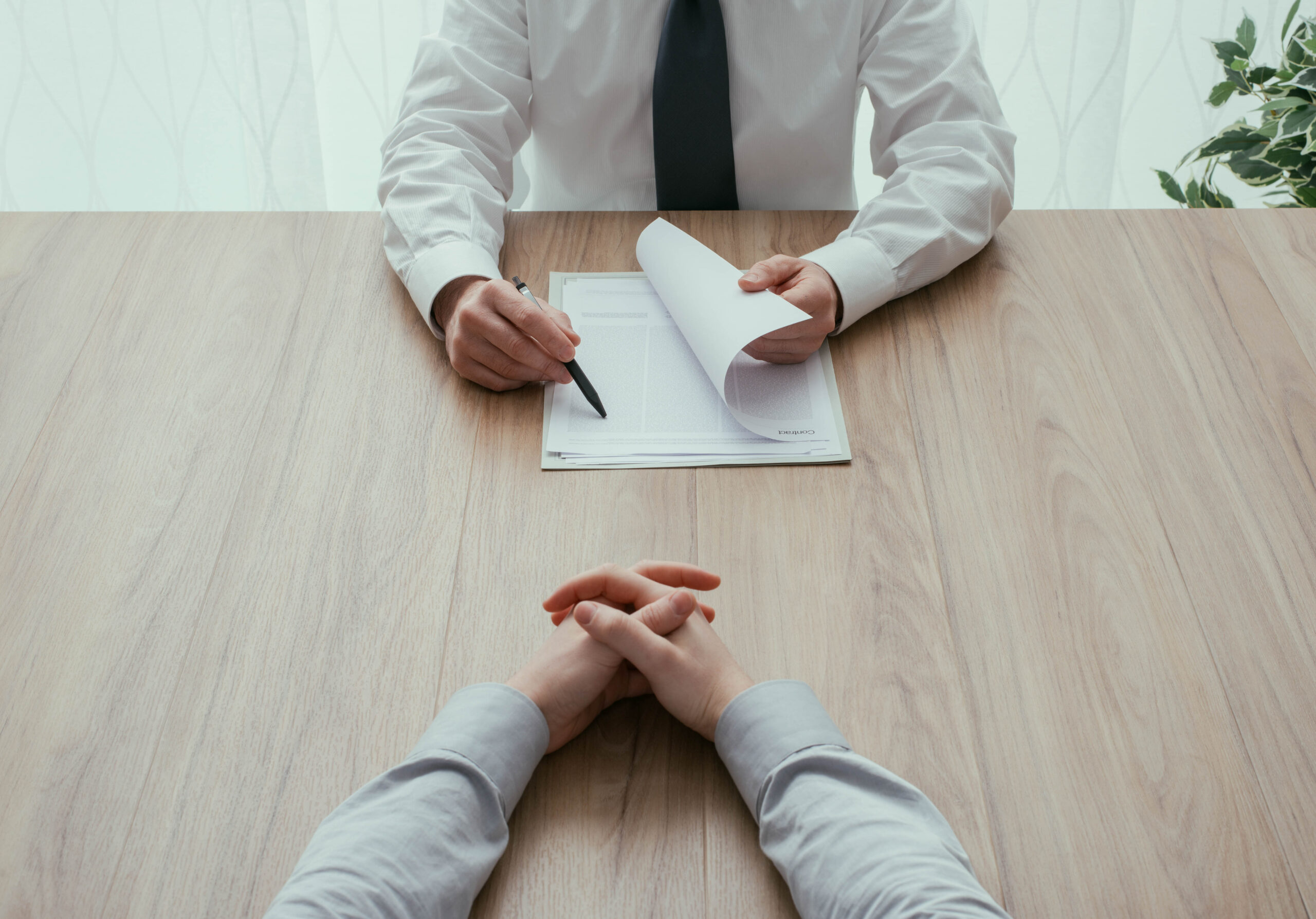
6 Fragen an unseren Senior Consultant Dominique
Heute möchten wir euch unseren IT-Berater und Entwickler Dominique vorstellen. Er ist seit November 2020 Teil unseres Teams und Spezialist in Java, Spring, Microsoft und Enterprise.
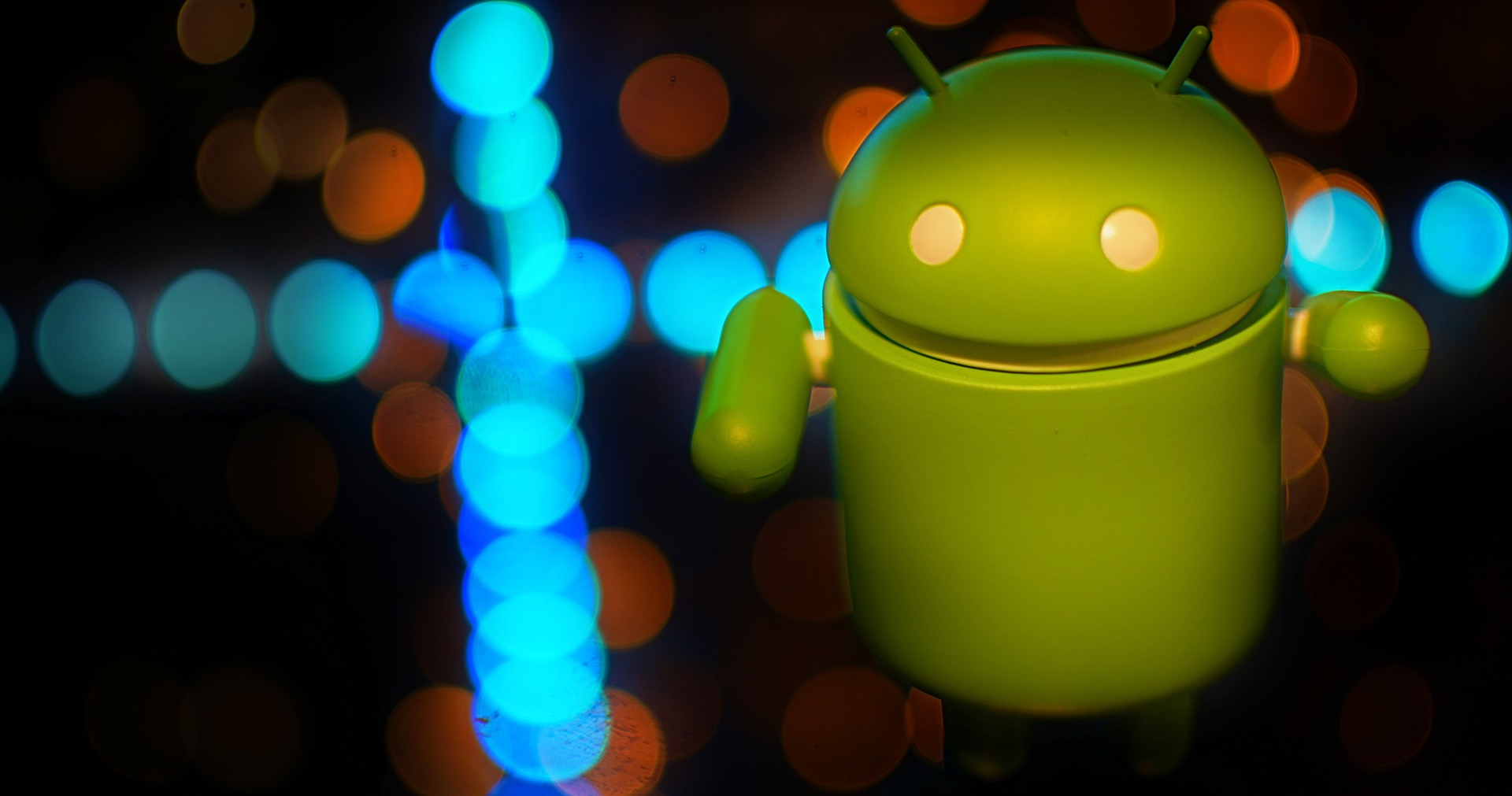
Repository in Android’s MVVM architecture
Repository is a class which purpose is to provide a clean API for accessing data. What that means is that the Repository can gather data from different data sources(different REST APIs, cache, local database storage) and it provides this data to the rest of the app.