Dependency Injection
What is Dependency Injection?
Each Object-oriented application consists of many classes that work together in order to solve a problem. However, when writing a complex application, application classes should be as independent as possible. DI helps in gluing these classes together and at the same time keeping them independent. Dependency injection is a technique whereby one object (or static method) supplies the dependencies of another object.
Pros
- increase the possibility to reuse classes
- test them independently of other classes while unit testing
Code with no DI vs code with DI
public class Computer {
private CPU cpu;
public Computer() {
this.cpu = new CPU();
}
}
Dependency between Computer and CPU.
public class Computer {
private CPU cpu;
public Computer(CPU cpu) {
this.cpu = cpu;
}
}
Dependency between Computer and CPU. Dependency is injected through class constructor (Computer should not worry about CPU implementation).
Types of DI
- Constructor-based dependency injection – used for mandatory dependencies
public Computer(CPU cpu) {
this.cpu = cpu;
}
- Setter-based dependency injection – used for optional dependencies
public Computer(CPU cpu) {
this.cpu = cpu;
}
- Property-based dependency injection – considered as bad practice!
@Autowired
private CPU cpu;
Letzte Beiträge
Share :
Share :
Weitere Beiträge
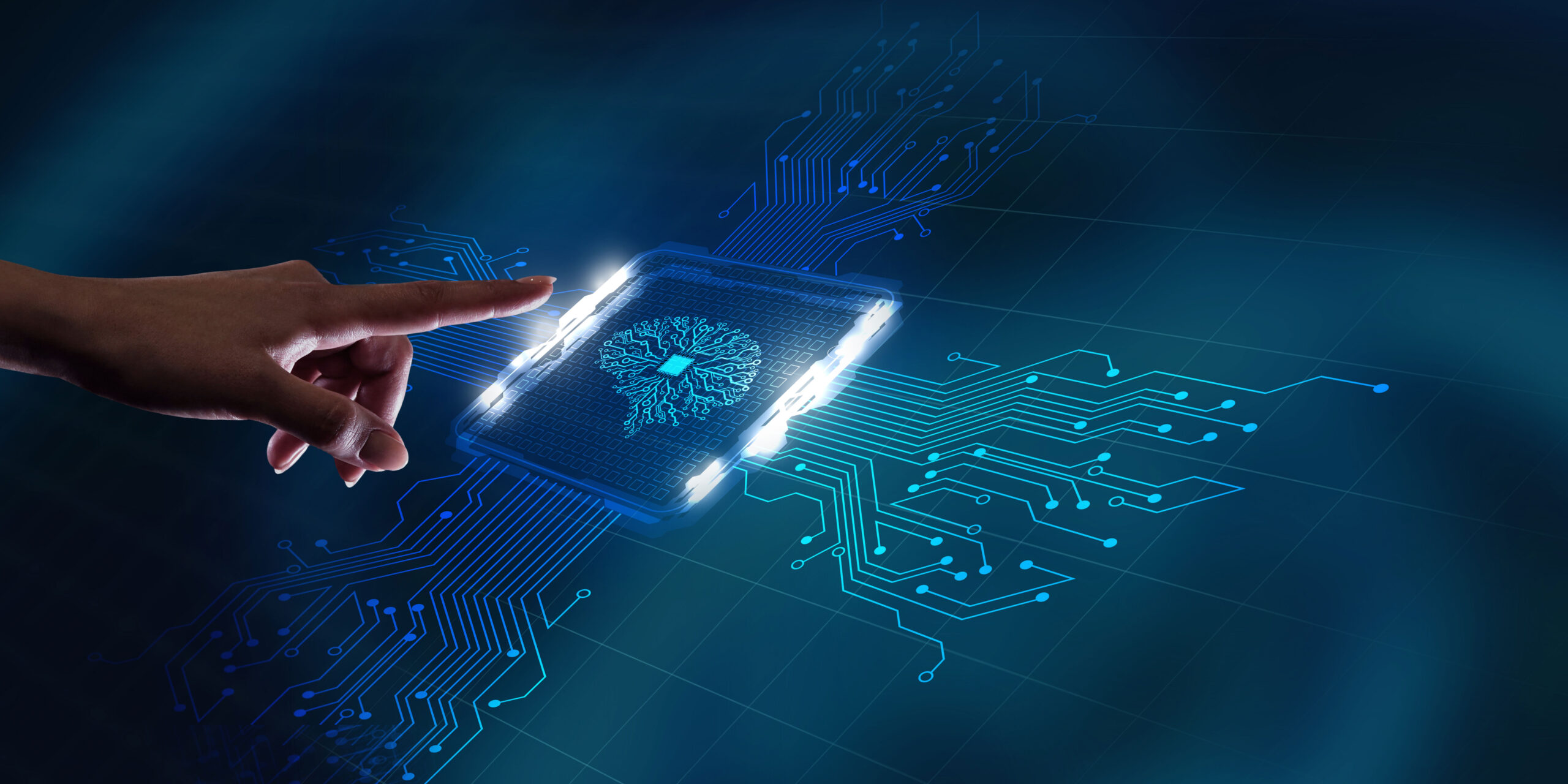
Machine learning concepts. Network training and evaluation
The neural network model consists of two layers – an LSTM layer and an output Dense layer. The reason for choosing an LSTM layer is the need to process sequences of time-related data
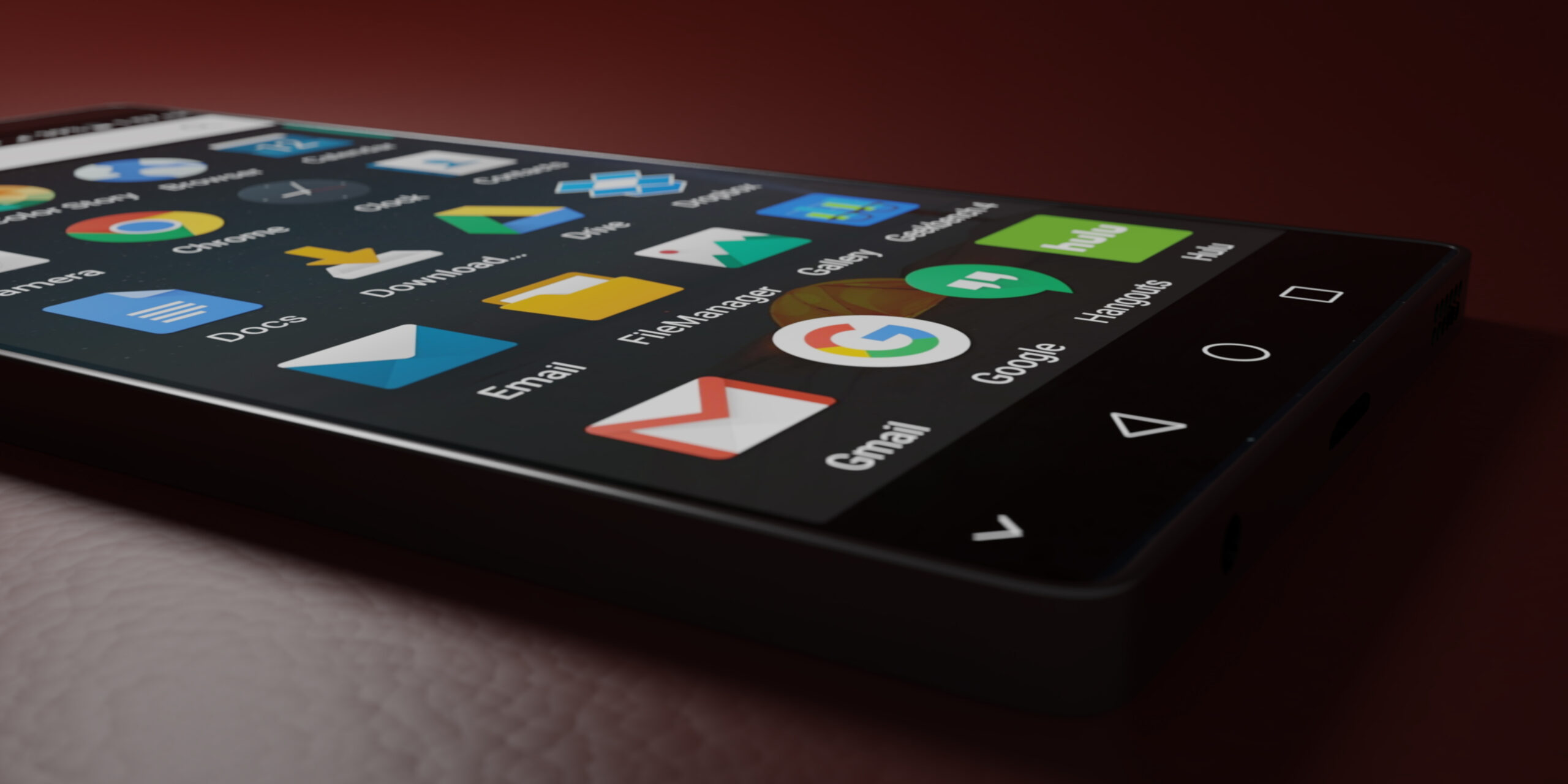
MVVM and its implementation in Android
Writing application code before thinking about an architecture is a really bad idea. Android programming does not make a difference. Everybody who starts creating Android apps begins doing so without following a specific architecture.
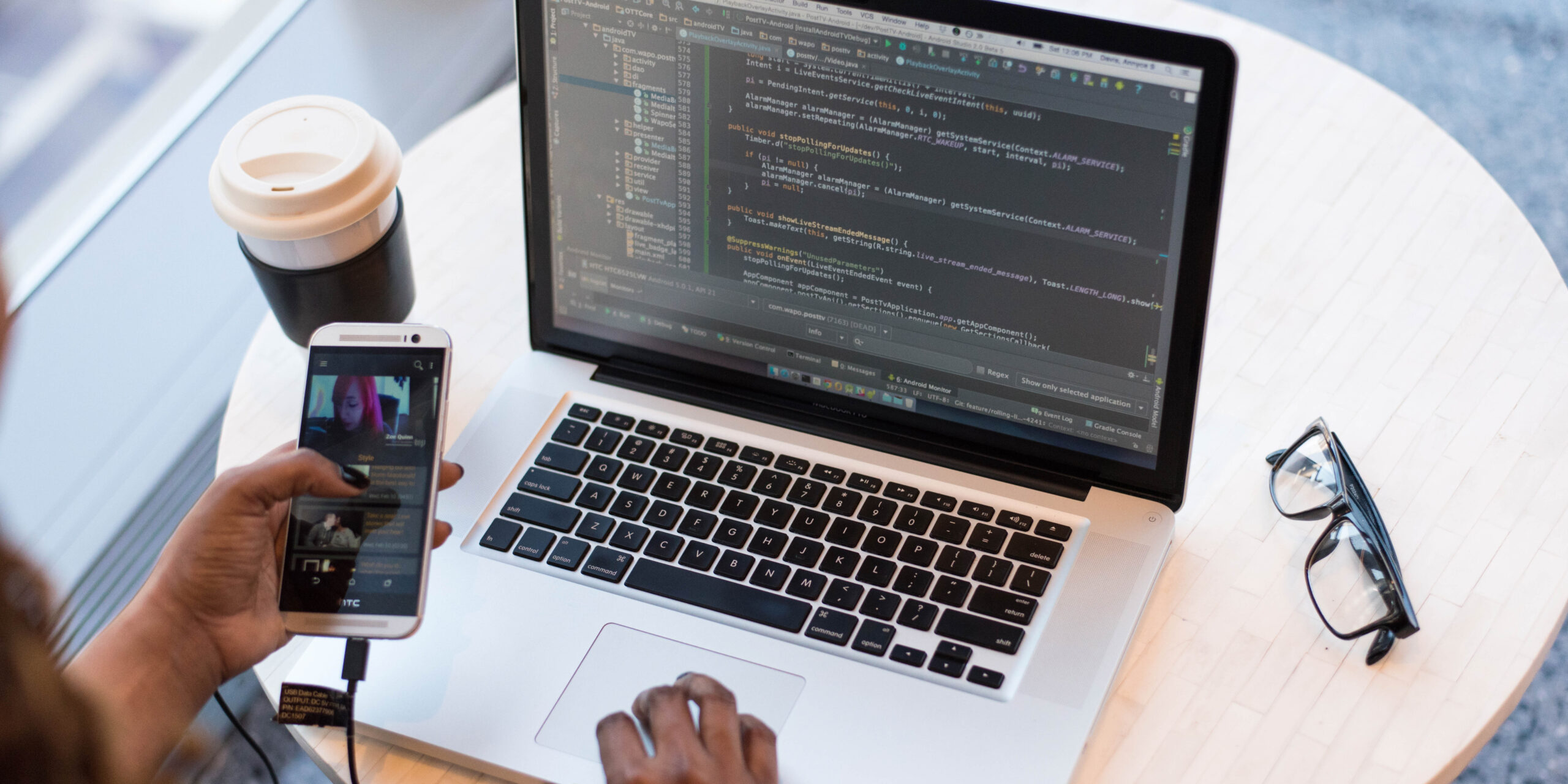
LiveData
LiveData is an observable data holder class. It is also lifecycle-aware, which means that it respects the lifecycle of the other app components, such as activities, fragments and services. It notifies only active observers, represented by the Observer class.