Missing Authorization header in Angular 7 HTTP response
Accessing an API back-end from Angular client may result in missing response headers. A common scenario is a missing Authorization header, containing the JSON Web Token (JWT) which is returned from the back-end service when the user logs in successfully. The solution to the problem is to expose the desired header in the back-end code (notice that the Authorization header is not exposed by default). In the case of a Spring Boot back-end, we need to add the following line of code:
response.addHeader("Access-Control-Expose-Headers", "Authorization");
@Override
protected void successfulAuthentication(HttpServletRequest request, HttpServletResponse response,
FilterChain chain, Authentication authResult) throws IOException, ServletException {
String token = JWT.create()
.withSubject(((User) authResult.getPrincipal()).getUsername())
.withExpiresAt(new Date(System.currentTimeMillis() + EXPIRATION_TIME))
.sign(HMAC512(SECRET.getBytes()));
response.addHeader("Access-Control-Expose-Headers", "Authorization");
response.addHeader(HEADER_STRING, TOKEN_PREFIX + token);
}
Letzte Beiträge
Share :
Share :
Weitere Beiträge
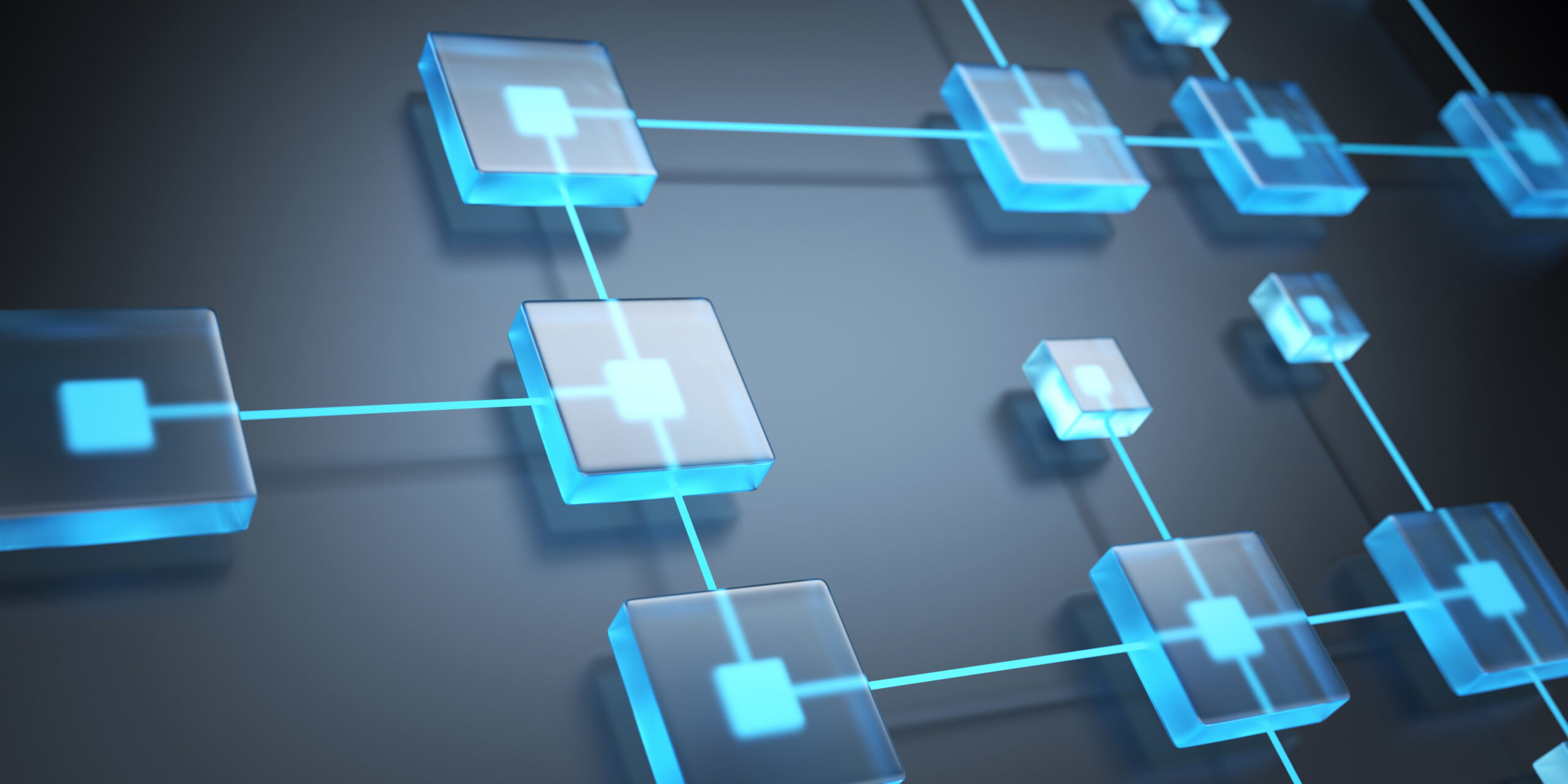
Implementing REST service architecture
In the previous article we got a sense of the REST architecture. In this article we will go through the steps of implementing the architecture. Our REST service will provide cryptocurrency predictions.
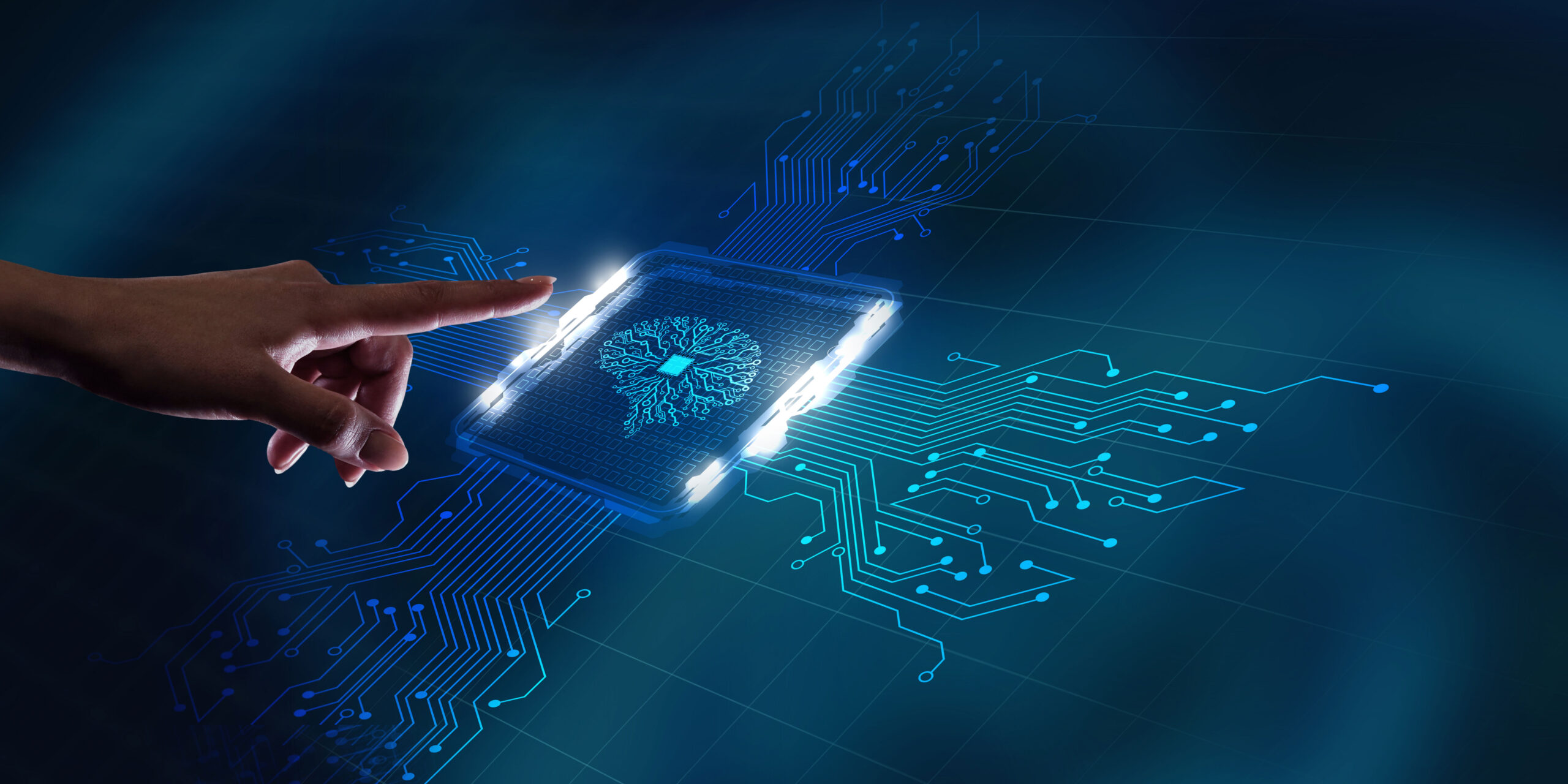
Machine learning concepts. Network training and evaluation
The neural network model consists of two layers – an LSTM layer and an output Dense layer. The reason for choosing an LSTM layer is the need to process sequences of time-related data
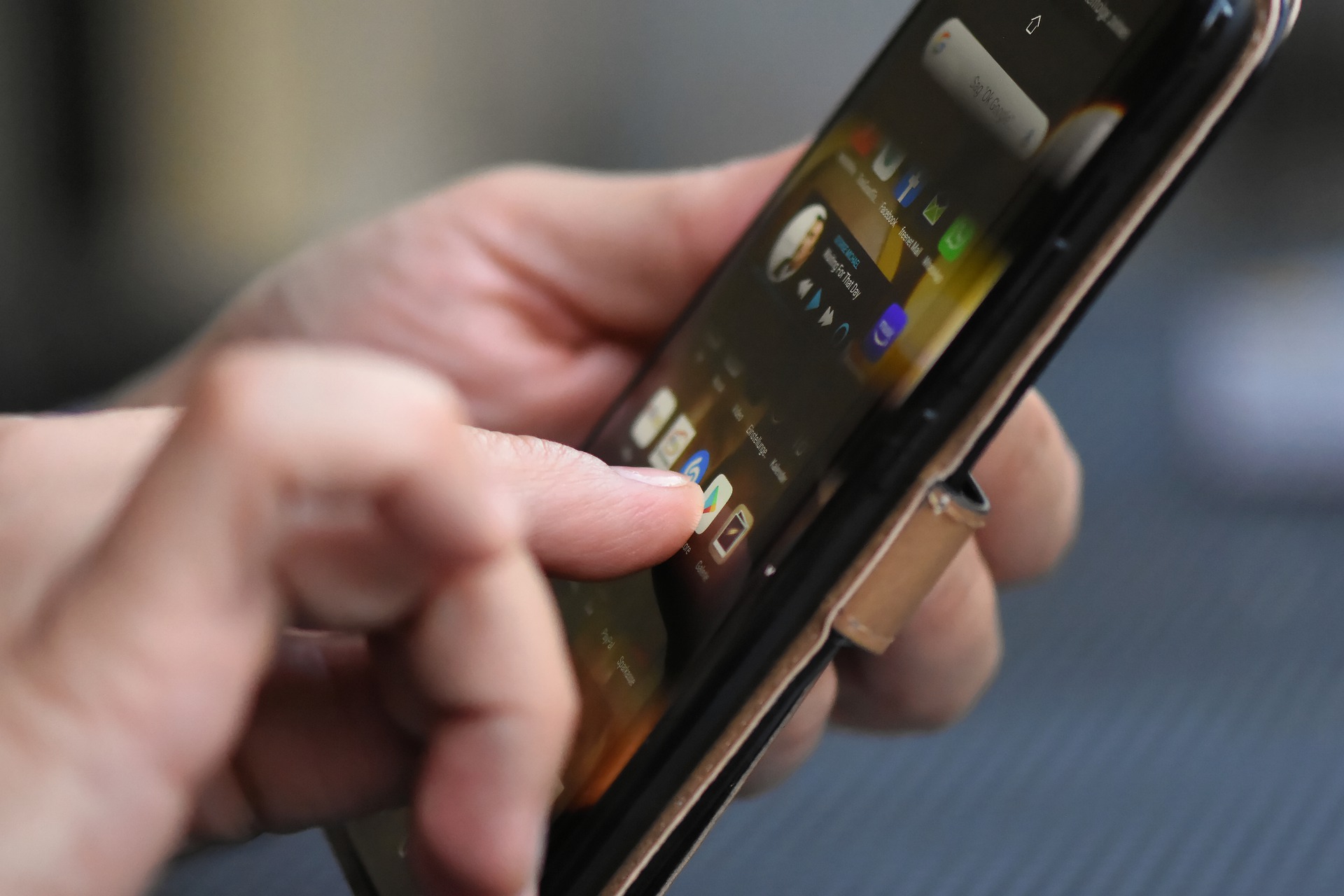
View Model. Example
In this article we will go through the steps of creating a simple game screen. We will make it the traditional way without using View Model and we will see why it is absolutely wrong to persist data in the View.